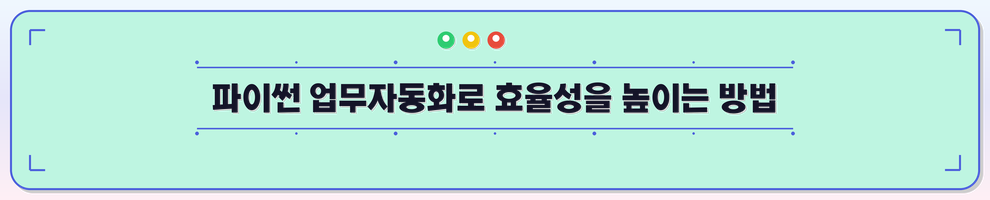
파이썬은 현대 업무 환경에서 반복적이고 시간 소모적인 작업을 자동화하는 데 탁월한 도구입니다. 초보자도 쉽게 배울 수 있는 문법과 다양한 라이브러리 덕분에, 파일 관리부터 데이터 처리, 웹 스크래핑까지 광범위한 작업을 자동화할 수 있습니다. 이 글에서는 실용적인 자동화 예제와 함께 필요한 기초 지식을 제공하여 업무 효율성을 크게 향상시킬 수 있는 방법을 안내합니다.
목차
파이썬 자동화의 이해
현대 비즈니스 환경에서 반복적인 작업은 시간과 리소스의 주요 소모원입니다. 파이썬(Python)은 이러한 작업을 자동화하여 업무 효율성을 크게 향상시킬 수 있는 강력한 프로그래밍 언어입니다.
파이썬이 자동화에 적합한 이유
파이썬이 업무 자동화에 널리 사용되는 이유는 다음과 같습니다:
- 간결하고 읽기 쉬운 문법: 영어와 유사한 구문으로 초보자도 쉽게 배울 수 있습니다.
- 풍부한 라이브러리 생태계: 거의 모든 작업을 위한 사전 제작된 도구가 존재합니다.
- 크로스 플랫폼 호환성: Windows, Mac, Linux 등 다양한 환경에서 작동합니다.
- 강력한 커뮤니티 지원: 문제 해결을 위한 광범위한 리소스가 있습니다.
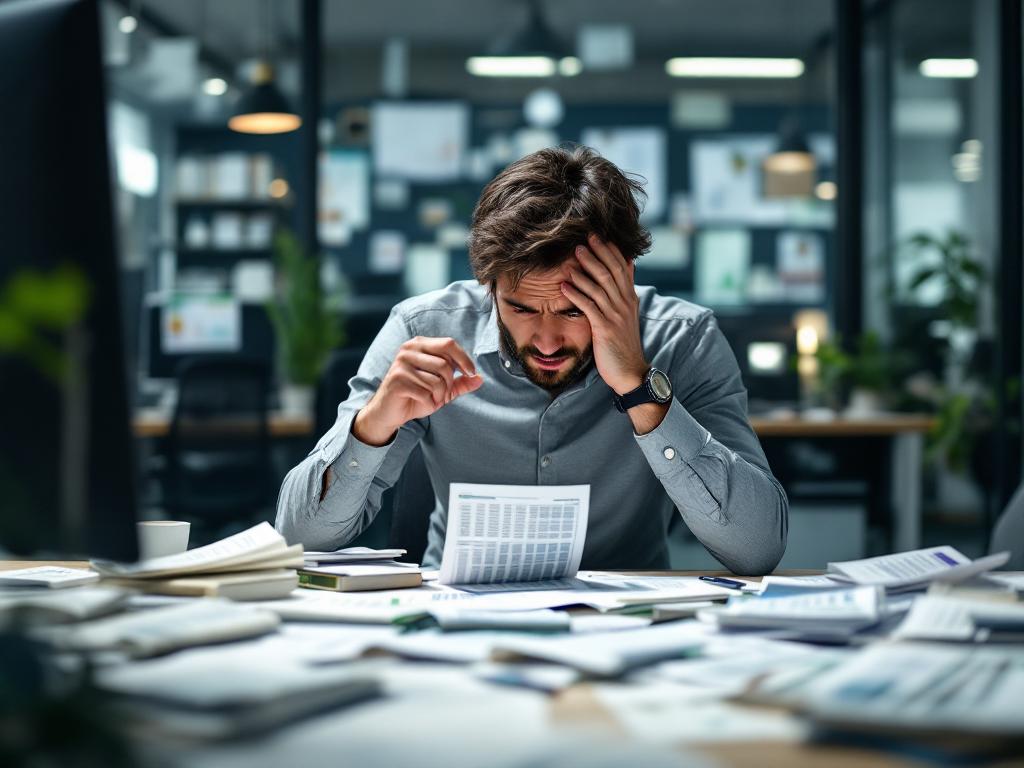
파이썬 자동화를 통해 해결할 수 있는 일반적인 업무 과제들은 다음과 같습니다:
- 대량의 파일 이름 변경 및 정리
- Excel 또는 CSV 파일의 데이터 처리 및 정리
- 웹사이트에서 정보 수집(웹 스크래핑)
- 정기 보고서 생성 및 이메일 전송
- API를 통한 서비스 자동화
- 반복적인 데이터 백업
파이썬 개발 환경 구축하기
파이썬 자동화의 첫 단계는 적절한 개발 환경을 구축하는 것입니다. 아래 과정을 따라 설치하세요:
파이썬 설치
- Python.org에서 최신 버전의 파이썬을 다운로드합니다.
- 설치 시 “Add Python to PATH” 옵션을 반드시 체크하세요.
- 설치가 완료되면 명령 프롬프트(또는 터미널)에서 다음 명령어로 확인합니다:
python --version
필수 라이브러리 설치
파이썬의 패키지 매니저 pip를 사용하여 필요한 라이브러리를 설치합니다:
pip install pandas numpy openpyxl requests beautifulsoup4 selenium
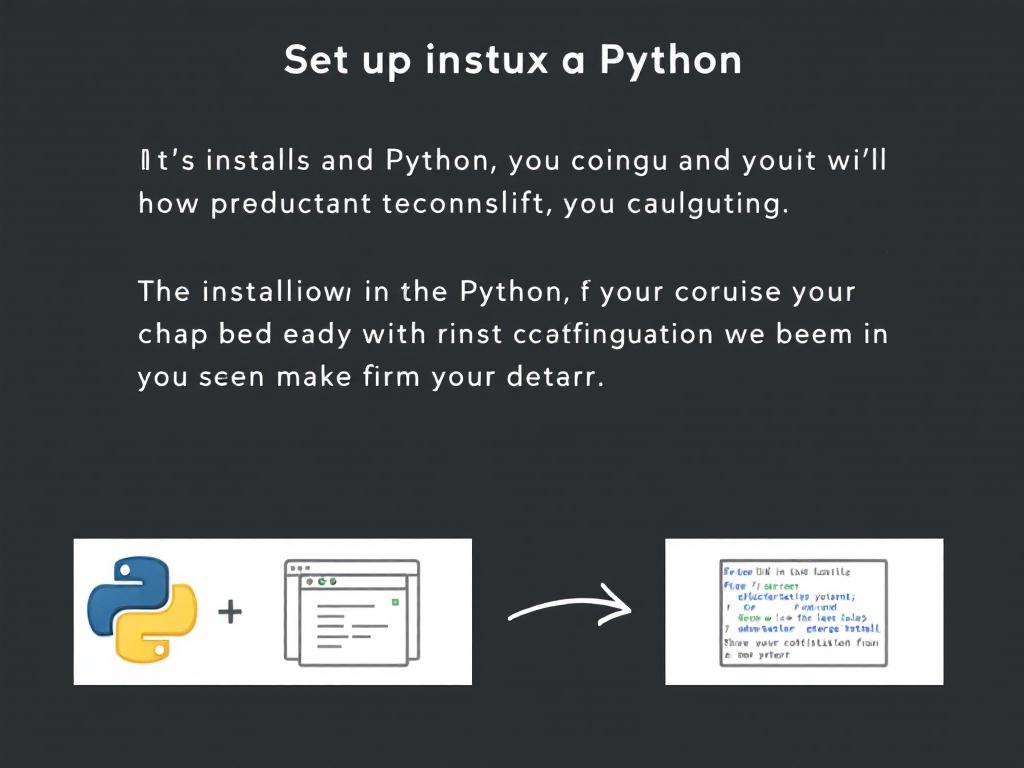
개발 환경 선택
효율적인 파이썬 코딩을 위해 적합한 개발 환경(IDE)을 선택하세요:
- VS Code: 가볍고 확장성 높은 무료 코드 에디터
- PyCharm: 강력한 기능을 갖춘 전문 Python IDE (무료 커뮤니티 버전 제공)
- Jupyter Notebook: 데이터 분석과 시각화에 적합한 대화형 환경
초보자라면 VS Code나 Jupyter Notebook으로 시작하는 것이 좋습니다.
파일 관리 자동화
파일 관리는 업무 자동화의 가장 일반적인 사용 사례입니다. 파이썬을 사용하면 수백, 수천 개의 파일도 쉽게 관리할 수 있습니다.
파일 정리 및 이름 변경
다음 스크립트는 지정된 폴더 내의 모든 파일을 확장자별로 분류합니다:
import os
import shutil
from pathlib import Path
def organize_files(directory):
# 각 파일 타입별 폴더 생성
file_types = {
'Images': ['.jpg', '.jpeg', '.png', '.gif', '.bmp'],
'Documents': ['.pdf', '.doc', '.docx', '.txt', '.xlsx', '.pptx'],
'Videos': ['.mp4', '.avi', '.mov', '.wmv'],
'Audio': ['.mp3', '.wav', '.flac', '.aac']
}
# 폴더 내 모든 파일 확인
for file in os.listdir(directory):
file_path = os.path.join(directory, file)
# 폴더는 건너뜀
if os.path.isdir(file_path):
continue
# 파일 확장자 확인
file_ext = os.path.splitext(file)[1].lower()
# 적절한 폴더로 파일 이동
for folder, extensions in file_types.items():
if file_ext in extensions:
folder_path = os.path.join(directory, folder)
# 필요시 폴더 생성
if not os.path.exists(folder_path):
os.makedirs(folder_path)
# 파일 이동
shutil.move(file_path, os.path.join(folder_path, file))
print(f"Moved {file} to {folder}")
break
# 사용 예시
organize_files("C:/Downloads")
일괄 파일 이름 변경
다음 스크립트는 특정 폴더 내 모든 이미지 파일의 이름을 형식에 맞게 변경합니다:
import os
import datetime
def rename_files(directory, prefix):
# 해당 폴더 내 모든 파일 검색
for i, filename in enumerate(os.listdir(directory)):
# 이미지 파일만 처리
if filename.endswith(('.jpg', '.jpeg', '.png')):
# 원래 파일의 경로
old_path = os.path.join(directory, filename)
# 현재 날짜를 파일명에 추가
today = datetime.datetime.now().strftime('%Y%m%d')
# 새 파일 이름 생성 (prefix_날짜_번호.확장자)
file_ext = os.path.splitext(filename)[1]
new_filename = f"{prefix}_{today}_{i+1:03d}{file_ext}"
# 새 파일 경로
new_path = os.path.join(directory, new_filename)
# 파일 이름 변경
os.rename(old_path, new_path)
print(f"Renamed: {filename} -> {new_filename}")
# 사용 예시
rename_files("C:/Photos", "vacation")
데이터 처리 자동화
엑셀 파일 처리, CSV 파일 병합, 데이터 정리 등의 작업은 파이썬으로 쉽게 자동화할 수 있습니다.
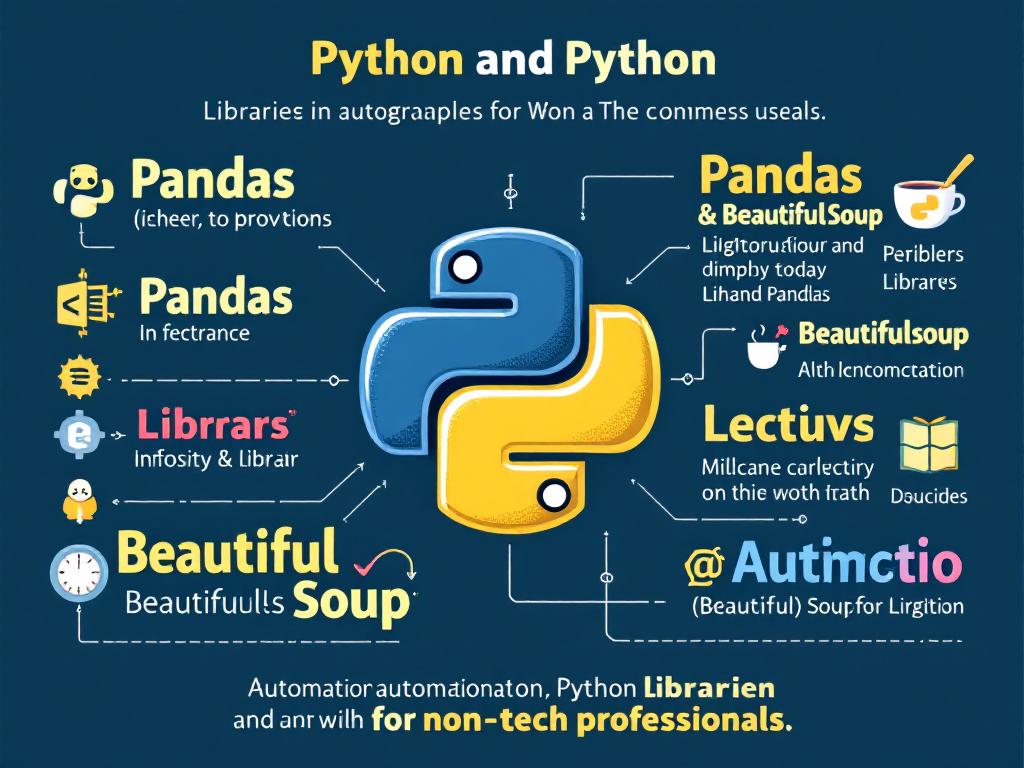
엑셀 파일 자동 처리
pandas 라이브러리를 사용하여 여러 엑셀 파일을 통합하고 분석하는 스크립트:
import pandas as pd
import glob
import os
def combine_excel_files(directory, output_file):
# 해당 디렉토리의 모든 엑셀 파일 검색
excel_files = glob.glob(os.path.join(directory, "*.xlsx"))
# 결과를 저장할 빈 데이터프레임 생성
combined_df = pd.DataFrame()
# 각 엑셀 파일을 순회하며 데이터 결합
for excel_file in excel_files:
# 현재 파일 로드
df = pd.read_excel(excel_file)
# 파일 이름을 새 열에 추가 (출처 추적)
file_name = os.path.basename(excel_file)
df['Source_File'] = file_name
# 결합 데이터프레임에 추가
combined_df = pd.concat([combined_df, df], ignore_index=True)
# 결과 저장
combined_df.to_excel(output_file, index=False)
print(f"Combined {len(excel_files)} files into {output_file}")
# 간단한 통계 반환
return {
"Total Files": len(excel_files),
"Total Rows": len(combined_df),
"Columns": combined_df.columns.tolist()
}
# 사용 예시
stats = combine_excel_files("C:/Monthly_Reports", "C:/Combined_Report.xlsx")
print(stats)
데이터 정리 및 변환
판매 데이터를 정리하고 분석하는 자동화 스크립트:
import pandas as pd
import matplotlib.pyplot as plt
def analyze_sales_data(file_path):
# 데이터 로드
df = pd.read_excel(file_path)
# 데이터 정리
# 결측치 처리
df.dropna(subset=['Sale_Amount'], inplace=True)
# 중복 제거
df.drop_duplicates(inplace=True)
# 날짜 형식 변환
df['Sale_Date'] = pd.to_datetime(df['Sale_Date'])
# 월별 컬럼 추가
df['Month'] = df['Sale_Date'].dt.strftime('%Y-%m')
# 월별 판매 합계 계산
monthly_sales = df.groupby('Month')['Sale_Amount'].sum().reset_index()
# 결과 시각화 및 저장
plt.figure(figsize=(12, 6))
plt.bar(monthly_sales['Month'], monthly_sales['Sale_Amount'])
plt.title('Monthly Sales')
plt.xlabel('Month')
plt.ylabel('Total Sales')
plt.xticks(rotation=45)
plt.tight_layout()
# 그래프 저장
plt.savefig('monthly_sales.png')
# 요약 보고서 생성
summary = {
'Total Sales': df['Sale_Amount'].sum(),
'Average Sale': df['Sale_Amount'].mean(),
'Best Month': monthly_sales.loc[monthly_sales['Sale_Amount'].idxmax(), 'Month'],
'Worst Month': monthly_sales.loc[monthly_sales['Sale_Amount'].idxmin(), 'Month']
}
# 정리된 데이터 저장
df.to_excel('cleaned_sales_data.xlsx', index=False)
monthly_sales.to_excel('monthly_sales_summary.xlsx', index=False)
return summary
# 사용 예시
results = analyze_sales_data('raw_sales_data.xlsx')
print(results)
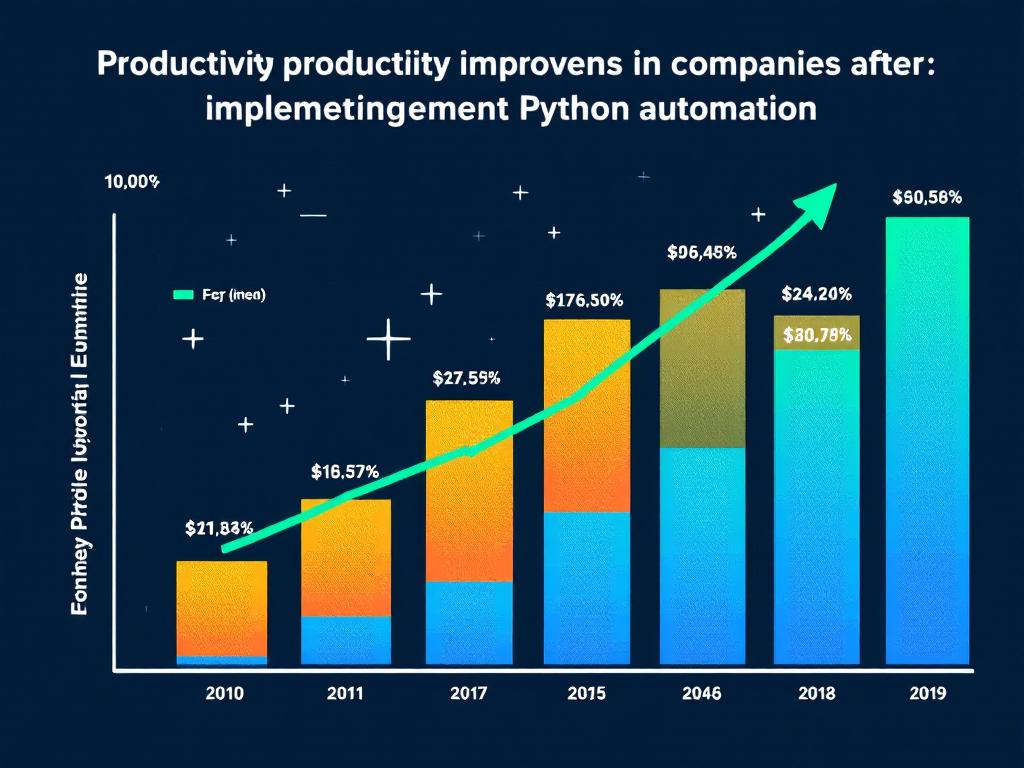
웹 자동화와 스크래핑
웹사이트에서 데이터를 수집하거나 반복적인 웹 작업을 자동화하는 것은 업무 효율성을 크게 높일 수 있습니다.
웹 스크래핑 기본
아래 코드는 Beautiful Soup을 사용하여 웹사이트의 뉴스 헤드라인을 수집합니다:
import requests
from bs4 import BeautifulSoup
import pandas as pd
from datetime import datetime
def scrape_news_headlines(url):
# 웹페이지 요청
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
# 응답 확인
if response.status_code != 200:
print(f"Error accessing the website: {response.status_code}")
return None
# 페이지 콘텐츠 파싱
soup = BeautifulSoup(response.text, 'html.parser')
# 뉴스 헤드라인 요소 찾기 (웹사이트에 따라 선택자가 다를 수 있음)
headlines = soup.select('h2.news-headline')
# 결과를 저장할 리스트
news_list = []
# 각 헤드라인 추출
for headline in headlines:
title = headline.text.strip()
# 링크가 있는 경우 추출
link_tag = headline.find('a')
link = link_tag['href'] if link_tag and link_tag.has_attr('href') else 'No link'
# 결과 저장
news_list.append({
'Title': title,
'Link': link,
'Date_Scraped': datetime.now().strftime('%Y-%m-%d')
})
# 데이터프레임으로 변환
news_df = pd.DataFrame(news_list)
# CSV 파일로 저장
filename = f"news_headlines_{datetime.now().strftime('%Y%m%d')}.csv"
news_df.to_csv(filename, index=False, encoding='utf-8-sig')
print(f"Scraped {len(news_list)} headlines and saved to {filename}")
return news_df
# 사용 예시
headlines_df = scrape_news_headlines('https://example-news-website.com')
웹 브라우저 자동화
Selenium을 사용하여 웹 브라우저 작업을 자동화하는 스크립트:
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from webdriver_manager.chrome import ChromeDriverManager
import time
import pandas as pd
def automate_web_search(search_terms):
# ChromeDriver 자동 설치 및 설정
service = Service(ChromeDriverManager().install())
driver = webdriver.Chrome(service=service)
results = []
try:
for term in search_terms:
# 검색 엔진으로 이동
driver.get('https://www.google.com')
# 검색어 입력 필드 찾기
search_box = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.NAME, 'q'))
)
# 검색어 입력 및 검색
search_box.clear()
search_box.send_keys(term)
search_box.submit()
# 검색 결과 로딩 대기
WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, 'search'))
)
# 검색 결과 수집
search_results = driver.find_elements(By.CSS_SELECTOR, 'div.g')
term_results = []
# 첫 5개 결과 추출
for i, result in enumerate(search_results[:5]):
try:
title_element = result.find_element(By.CSS_SELECTOR, 'h3')
title = title_element.text
link_element = result.find_element(By.CSS_SELECTOR, 'a')
link = link_element.get_attribute('href')
term_results.append({
'Search_Term': term,
'Rank': i + 1,
'Title': title,
'Link': link
})
except Exception as e:
print(f"Error extracting result {i+1} for {term}: {e}")
results.extend(term_results)
# 과도한 요청 방지를 위한 대기
time.sleep(2)
finally:
# 브라우저 종료
driver.quit()
# 결과를 데이터프레임으로 변환
results_df = pd.DataFrame(results)
# 파일로 저장
results_df.to_excel('search_results.xlsx', index=False)
return results_df
# 사용 예시
search_terms = ['python automation', 'work productivity tools', 'data processing python']
results = automate_web_search(search_terms)
이메일과 보고서 자동화
이메일 전송과 보고서 생성을 자동화하면 정기적인 업무 커뮤니케이션을 크게 간소화할 수 있습니다.
자동 이메일 발송
파이썬으로 이메일을 자동 발송하는 스크립트:
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.application import MIMEApplication
import pandas as pd
from datetime import datetime
import os
def send_automated_emails(excel_file, email_col, name_col, subject, template, sender_email, sender_password, attachment_path=None):
# 이메일 데이터 로드
df = pd.read_excel(excel_file)
# SMTP 서버 설정 (Gmail 예시)
smtp_server = 'smtp.gmail.com'
smtp_port = 587
# SMTP 서버 연결
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls() # TLS 보안 시작
try:
# 로그인
server.login(sender_email, sender_password)
# 성공/실패 카운트
success_count = 0
failed_count = 0
failed_emails = []
# 각 수신자에게 이메일 발송
for index, row in df.iterrows():
recipient_email = row[email_col]
recipient_name = row[name_col]
# 이메일이 유효한지 확인
if pd.isna(recipient_email) or '@' not in recipient_email:
print(f"Skipping invalid email: {recipient_email}")
failed_count += 1
failed_emails.append(f"{recipient_name}: Invalid email")
continue
# 메시지 생성
msg = MIMEMultipart()
msg['From'] = sender_email
msg['To'] = recipient_email
msg['Subject'] = subject
# 이메일 템플릿에 개인화된 내용 추가
email_body = template.replace('{name}', recipient_name)
email_body = email_body.replace('{date}', datetime.now().strftime('%Y-%m-%d'))
# HTML 메시지 첨부
msg.attach(MIMEText(email_body, 'html'))
# 첨부 파일이 있는 경우
if attachment_path and os.path.exists(attachment_path):
with open(attachment_path, 'rb') as file:
attachment = MIMEApplication(file.read(), Name=os.path.basename(attachment_path))
attachment['Content-Disposition'] = f'attachment; filename="{os.path.basename(attachment_path)}"'
msg.attach(attachment)
try:
# 이메일 발송
server.send_message(msg)
print(f"Email sent to {recipient_name} ({recipient_email})")
success_count += 1
# 너무 많은 이메일을 한 번에 보내지 않도록 잠시 대기
if index % 10 == 0 and index > 0:
time.sleep(5)
except Exception as e:
print(f"Failed to send email to {recipient_email}: {e}")
failed_count += 1
failed_emails.append(f"{recipient_name} ({recipient_email}): {str(e)}")
# 결과 요약
results = {
'Total': len(df),
'Success': success_count,
'Failed': failed_count,
'Failed_List': failed_emails
}
return results
finally:
# SMTP 연결 종료
server.quit()
# 이메일 HTML 템플릿 예시
email_template = """
안녕하세요, {name}님!
이 달의 업무 보고서를 첨부합니다.
오늘 날짜: {date}
문의사항이 있으시면 언제든 연락주세요.
감사합니다.
"""
# 사용 예시 (실제 사용 시 보안 정보 보호 필요)
# send_automated_emails(
# 'contacts.xlsx',
# 'Email',
# 'Name',
# '월간 보고서',
# email_template,
# 'your_email@gmail.com',
# 'your_app_password', # Gmail App 비밀번호 사용
# 'monthly_report.pdf'
# )
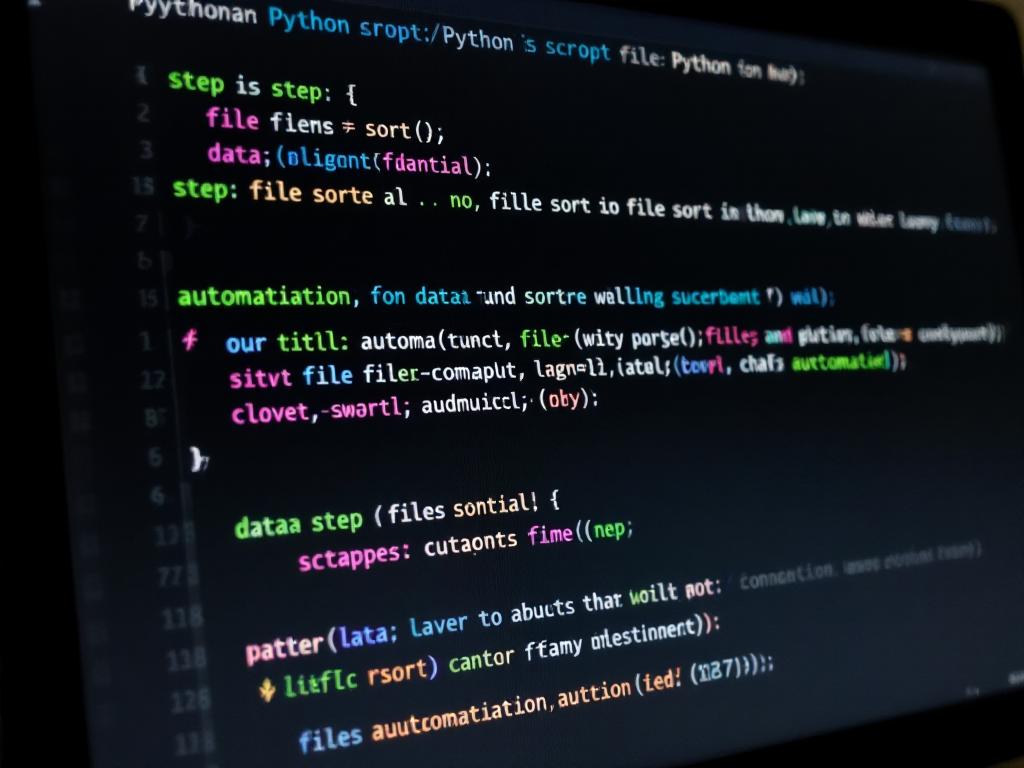
자동 보고서 생성
데이터 분석 결과를 PDF 보고서로 자동 생성하는 스크립트:
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
from reportlab.lib.pagesizes import letter
from reportlab.platypus import SimpleDocTemplate, Paragraph, Spacer, Image, Table, TableStyle
from reportlab.lib.styles import getSampleStyleSheet, ParagraphStyle
from reportlab.lib import colors
from reportlab.lib.units import inch
import io
import os
from datetime import datetime
def generate_sales_report(data_file, output_file):
# 데이터 로드
df = pd.read_excel(data_file)
# 날짜 열이 있다면 datetime으로 변환
if 'Date' in df.columns:
df['Date'] = pd.to_datetime(df['Date'])
df['Month'] = df['Date'].dt.strftime('%Y-%m')
# 시각화를 위한 이미지들 생성
plt.figure(figsize=(10, 6))
# 1. 월별 판매량 그래프
monthly_sales = df.groupby('Month')['Sales'].sum().reset_index()
plt.subplot(2, 2, 1)
sns.barplot(data=monthly_sales, x='Month', y='Sales')
plt.title('Monthly Sales')
plt.xticks(rotation=45)
plt.tight_layout()
# 2. 제품별 판매 분포 파이차트
plt.subplot(2, 2, 2)
product_sales = df.groupby('Product')['Sales'].sum()
plt.pie(product_sales, labels=product_sales.index, autopct='%1.1f%%')
plt.title('Sales by Product')
plt.tight_layout()
# 3. 지역별 판매량 막대 그래프
plt.subplot(2, 1, 2)
region_sales = df.groupby('Region')['Sales'].sum().sort_values(ascending=False)
sns.barplot(x=region_sales.index, y=region_sales.values)
plt.title('Sales by Region')
plt.tight_layout()
# 이미지를 임시 파일로 저장
chart_filename = 'temp_sales_chart.png'
plt.savefig(chart_filename, dpi=300, bbox_inches='tight')
plt.close()
# PDF 보고서 생성
doc = SimpleDocTemplate(output_file, pagesize=letter)
story = []
# 스타일 설정
styles = getSampleStyleSheet()
title_style = styles['Heading1']
heading_style = styles['Heading2']
normal_style = styles['Normal']
# 사용자 정의 스타일
custom_normal = ParagraphStyle(
'CustomNormal',
parent=styles['Normal'],
fontSize=10,
leading=14,
)
# 제목 추가
report_title = f"Sales Performance Report - {datetime.now().strftime('%B %Y')}"
story.append(Paragraph(report_title, title_style))
story.append(Spacer(1, 0.25*inch))
# 요약 통계 추가
story.append(Paragraph("Executive Summary", heading_style))
story.append(Spacer(1, 0.1*inch))
summary_text = f"""
This report presents the sales performance analysis for the period ending {datetime.now().strftime('%B %d, %Y')}.
Total Sales: ${df['Sales'].sum():,.2f}
Average Monthly Sales: ${df.groupby('Month')['Sales'].sum().mean():,.2f}
Best Performing Product: {product_sales.idxmax()} (${product_sales.max():,.2f})
Best Performing Region: {region_sales.index[0]} (${region_sales.values[0]:,.2f})
"""
story.append(Paragraph(summary_text, custom_normal))
story.append(Spacer(1, 0.2*inch))
# 차트 이미지 추가
story.append(Paragraph("Sales Visualization", heading_style))
story.append(Spacer(1, 0.1*inch))
img = Image(chart_filename, width=6.5*inch, height=4*inch)
story.append(img)
story.append(Spacer(1, 0.2*inch))
# 표 데이터 추가
story.append(Paragraph("Detailed Sales Data", heading_style))
story.append(Spacer(1, 0.1*inch))
# 상위 10개 판매 데이터 표시
top_sales = df.sort_values('Sales', ascending=False).head(10)
# 테이블 데이터 준비
table_data = [['Date', 'Product', 'Region', 'Sales']] # 헤더 행
for _, row in top_sales.iterrows():
sale_date = row['Date'].strftime('%Y-%m-%d') if isinstance(row['Date'], pd.Timestamp) else row['Date']
table_data.append([
sale_date,
row['Product'],
row['Region'],
f"${row['Sales']:,.2f}"
])
# 테이블 스타일 설정
table = Table(table_data, colWidths=[1.2*inch, 2*inch, 1.5*inch, 1*inch])
table.setStyle(TableStyle([
('BACKGROUND', (0, 0), (-1, 0), colors.lightgreen),
('TEXTCOLOR', (0, 0), (-1, 0), colors.black),
('ALIGN', (0, 0), (-1, -1), 'CENTER'),
('FONTNAME', (0, 0), (-1, 0), 'Helvetica-Bold'),
('BOTTOMPADDING', (0, 0), (-1, 0), 12),
('BACKGROUND', (0, 1), (-1, -1), colors.white),
('GRID', (0, 0), (-1, -1), 1, colors.black),
]))
story.append(table)
# PDF 생성
doc.build(story)
# 임시 파일 삭제
if os.path.exists(chart_filename):
os.remove(chart_filename)
print(f"Report successfully generated: {output_file}")
return output_file
# 사용 예시
# generate_sales_report('sales_data.xlsx', 'Sales_Performance_Report.pdf')
자동화 모범 사례와 주의사항
효율적이고 안전한 자동화를 위한 주요 모범 사례와 주의사항을 알아봅시다.
자동화 모범 사례
- 코드 재사용성 높이기: 기능별로 함수를 분리하고, 설정 값은 외부에서 쉽게 변경할 수 있도록 설계하세요.
- 오류 처리 포함하기: 예외 처리를 통해 스크립트가 중간에 중단되지 않도록 하세요.
- 로깅(logging) 사용하기: 자동화 과정에서 무슨 일이 일어나는지 기록하고 문제 해결에 활용하세요.
- 변경 전 백업하기: 파일을 수정하거나 이동하기 전에 항상 원본 데이터를 백업하세요.
- 단계적으로 테스트하기: 작은 데이터 셋에서 먼저 테스트한 후 전체 데이터에 적용하세요.
주의사항
자동화 스크립트 사용 시 다음 사항에 유의하세요:
- 중요한 데이터는 항상 백업하세요: 자동화 스크립트에 버그가 있으면 많은 데이터를 한 번에 손상시킬 수 있습니다.
- API 사용 한도에 주의하세요: 웹 스크래핑이나 API 호출 시 서버의 사용 제한을 확인하고 준수하세요.
- 보안 정보 보호하기: 비밀번호나 API 키는 코드에 직접 포함하지 말고 환경 변수나 별도 설정 파일을 사용하세요.
- 무한 루프에 빠지지 않도록 하세요: 자동화 스크립트에 적절한 중단 조건을 추가하세요.
- 리소스 사용량 모니터링: 대량의 데이터를 처리할 때는 메모리 사용량을 주의 깊게 모니터링하세요.
아래 코드는 자동화 스크립트를 위한 기본적인 로깅과 오류 처리 템플릿입니다:
import logging
import os
import time
from datetime import datetime
def setup_logging(log_file=None):
"""로깅 설정"""
if log_file is None:
log_file = f"automation_{datetime.now().strftime('%Y%m%d_%H%M%S')}.log"
# 로그 디렉토리 확인
log_dir = os.path.dirname(log_file)
if log_dir and not os.path.exists(log_dir):
os.makedirs(log_dir)
# 로그 설정
logging.basicConfig(
level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s',
handlers=[
logging.FileHandler(log_file),
logging.StreamHandler() # 콘솔에도 출력
]
)
return logging.getLogger()
def safe_automation_template():
"""안전한 자동화를 위한 템플릿 함수"""
logger = setup_logging('logs/automation.log')
start_time = time.time()
logger.info("자동화 작업 시작")
try:
# 1. 데이터 백업
logger.info("데이터 백업 중...")
# 백업 코드 작성
# 2. 주요 작업 수행
logger.info("주요 작업 수행 중...")
# 여기에 실제 자동화 코드 작성
# 3. 결과 검증
logger.info("결과 검증 중...")
# 검증 코드 작성
execution_time = time.time() - start_time
logger.info(f"작업 완료! 실행 시간: {execution_time:.2f}초")
return True
except Exception as e:
logger.error(f"오류 발생: {str(e)}", exc_info=True)
# 오류 발생 시 원상복구 작업
logger.info("원상복구 시도 중...")
# 복구 코드 작성
execution_time = time.time() - start_time
logger.info(f"작업 실패! 실행 시간: {execution_time:.2f}초")
return False
# 사용 예시
if __name__ == "__main__":
success = safe_automation_template()
print(f"자동화 작업 {'성공' if success else '실패'}")
자주 묻는 질문
Q: 프로그래밍 경험이 전혀 없어도 파이썬 자동화를 배울 수 있나요?
A: 네, 가능합니다. 파이썬은 초보자에게 가장 친화적인 프로그래밍 언어 중 하나입니다. 온라인 무료 강좌나 “업무 자동화를 위한 파이썬” 같은 입문서로 시작해보세요. 처음에는 간단한 스크립트부터 시작하여 점진적으로 학습하는 것이 효과적입니다.
Q: 자동화할 가치가 있는 업무를 어떻게 식별할 수 있나요?
A: 일반적으로 다음과 같은 작업이 자동화에 적합합니다: 1) 반복적으로 수행하는 작업, 2) 패턴이 명확한 작업, 3) 오류 가능성이 높은 수동 작업, 4) 많은 시간이 소요되는 데이터 처리 작업. 일주일 동안 업무를 기록하고 반복되는 패턴을 찾아보세요.
Q: 웹 스크래핑이 합법적인가요?
A: 웹 스크래핑의 합법성은 대상 웹사이트의 이용 약관, 스크래핑 방법, 데이터 사용 목적에 따라 달라집니다. 일반적으로 robots.txt 파일을 존중하고, 서버에 과도한 부하를 주지 않으며, 저작권이 있는 콘텐츠의 무단 사용을 피하는 것이 중요합니다. 상업적 용도로 스크래핑을 계획한다면 법률 자문을 구하는 것이 좋습니다.
Q: 파이썬 자동화 스크립트를 정기적으로 실행하는 방법은 무엇인가요?
A: 운영체제에 따라 다양한 방법이 있습니다. Windows에서는 작업 스케줄러를, Mac/Linux에서는 cron을 사용할 수 있습니다. 클라우드 환경에서는 AWS Lambda, Google Cloud Functions 같은 서비스와 함께 스케줄러를 사용할 수 있습니다. 또한 파이썬 라이브러리인 schedule이나 APScheduler를 사용하여 코드 내에서 직접 일정을 관리할 수도 있습니다.
Q: 자동화 스크립트의 성능을 개선하려면 어떻게 해야 하나요?
A: 성능 개선을 위한 몇 가지 방법은 다음과 같습니다: 1) 병렬 처리를 위해 multiprocessing 또는 threading 모듈 사용, 2) 대량 데이터 처리에 NumPy, Pandas 같은 최적화된 라이브러리 활용, 3) 데이터베이스 작업에 일괄 처리(batch processing) 적용, 4) 불필요한 I/O 작업 최소화, 5) 코드 프로파일링을 통해 병목 지점 식별 및 개선. 실행 시간이 오래 걸리는 스크립트의 경우 이러한 최적화가 큰 차이를 만들 수 있습니다.
Q: 자동화 스크립트를 다른 사람들과 안전하게 공유하려면 어떻게 해야 하나요?
A: 스크립트 공유 시 다음 사항에 유의하세요: the 1) 민감한 정보(비밀번호, API 키 등)를 코드에서 분리하여 환경 변수나 별도의 설정 파일로 관리, 2) 명확한 문서화와 주석 추가, 3) 필요한 라이브러리와 버전을 requirements.txt 파일에 명시, 4) 오류 처리 체계 구축, 5) GitHub 등을 통해 공유하는 경우 .gitignore 파일을 사용하여 민감한 파일을 제외, 6) 가능하다면 코드를 패키지화하여 설치 및 사용 방법 간소화.